The traditional first program is that which says “Hello World”. This is a hoary staple
of all computer education, the "Iron Man" of programming, with no logic other than a one-line output.
It is the simplest of code, yet it contains all the elements you will need to run your program.
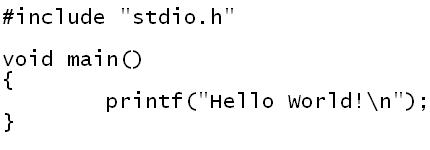
Let's look at it line by line.
————————————>

As discussed, you will be using a compiler to convert your C code to binary format.
Therefore, you will see some things that are specific to the compiler. These are called "directives". The "#include"
is a directive for the compiler to find a "header file" and add it to your code.
Header files are interfaces to other code. This is important because programs build on other programs.
Your program will be using a previously written and compiled collection of functions. In the C environment, these collections are
called libraries. (In the Java language environment, by comparison, they are called jars.) Header files describe the library's data structures and function calls.
Without these header files to interface with their code, the compiler won't recognize their function calls in your code.
In this case, the compiler needs to understand the function "printf", which is called in the "main" function.
The "#include" directive gets the header file "stdio.h" and compiles it with your own code. (In case you were wondering,
"stdio.h" is an abbreviation for "standard input/output".)
————————————>

Every C program needs a "main" function. Without a call to the "main" function,
the program would have nowhere to go.
Therefore, it is the one essential line in almost every C program. If you've ever seen other languages, you will have seen
a "main" function before, because many of those other languages and scripting platforms are derived from C.
Note the first token "void". This is the "returned data type" (more on returned data types in a second).
"void" is a special data type, which means "nothing at all." It may seem hopelessly strict to have to say that, but that's how it is.
————————————>

This curly bracket makes a "block" of code. That is, code can be partitioned into separate parts, called blocks,
which help structure your logic.
In the C language, blocks are "delimited" (i.e., started and stopped) with the curly brackets '{' and '}'. In this example, the only block of
code is the "main" function itself.
————————————>

This is our first "function call". Let's take a moment to discuss this concept. It's very important.
Unlike the passive nature of other data, a function does something active. A function is code which is written to execute.
It will take input through its parameters, and return output of a certain data type. The description of this function is called a "prototype".
These function prototypes are declared in those header files we discussed. When you compile your program, it will link with the libraries,
and the function name will become a pointer to the executable code in the library.
"printf" is a function. Like other functions, its input arguments are contained within the parentheses which follow it.
This particular function takes one argument, the "Hello World!\n" string (more on strings later). When you write this function into your program,
with the parentheses and necessary arguments, the compiler assumes that you intend to execute it.
This is known as "calling", or "invoking" the function.
Please note the '\n' character in the string argument. You will see this often. A character preceded by a
backslash '\' is called an "escape" sequence. The backslash "escapes" the ASCII character that follows it, which, in this case,
is the 'n' character. In its place is an invisible character, which, in this case, is a new line.
Other escape sequences include the tab character '\t' and the null character '\0'. (The null character ends the string.)
————————————>

With this end curly bracket, the "main" function is over. Congratulations! You have written your first C program.
Name this file something like "HelloWorld.c". (The ".c" suffix identifies a C program.) When you compile it,
you will have an "executable" file called "HelloWorld.exe". You can run this from the command line like this:

Your output will look like this:
